Loading data into Pyleoclim objects#
To simplify the remainder of the examples in this notebook, we’ll load the LiPD data into pickle objects which can be stored and easily loaded later. These are python native data objects, so we’re essentially trading the flexibility of the LiPD files for the convenience of pickle files.
import pickle
import os
from tqdm import tqdm
import pyleoclim as pyleo
from pylipd.lipd import LiPD
Make a directory for the pickle files if it doesn’t yet exist:
pickle_dir = '../../data/pickle'
if not os.path.exists(pickle_dir):
os.makedirs(pickle_dir)
Loading and processing the data:
data_path = '../../data/LiPD/full'
D = LiPD()
D.load_from_dir(data_path)
lipd_records = D.get_all_dataset_names()
series_dict = {}
processed_series_dict = {}
ens_dict = {}
processed_ens_dict = {}
holocene_bounds = (0,10000)
for record in tqdm(lipd_records):
D = LiPD()
D.load(os.path.join(data_path,f'{record}.lpd'))
df = D.get_timeseries_essentials().iloc[0]
series = pyleo.GeoSeries(
time = df['time_values'],
value=df['paleoData_values'],
time_name = 'Age',
time_unit = 'yrs BP',
value_name = r'$\delta^{18} O$',
value_unit = u'‰',
label=record,
lat = df['geo_meanLat'],
lon=df['geo_meanLon'],
archiveType='speleothem',
dropna=False,
verbose = False
)
if record == 'Qunf.Oman.2023': # Removing a large hiatus from the Qunf record
series = series.slice((2500,10000))
processed_series = series.slice(holocene_bounds).interp().standardize().detrend(method='savitzky-golay')
series_dict[record] = series
processed_series_dict[record] = processed_series
ens_df = D.get_ensemble_tables().iloc[0]
ens_series_list = []
processed_ens_series_list = []
for i in range(1000): #We know there are 1000 ensemble members
ens_series = pyleo.GeoSeries(
time = ens_df['ensembleVariableValues'].T[i],
value= df['paleoData_values'],
time_name = 'Age',
time_unit = 'yrs BP',
value_name = r'$\delta^{18} O$',
value_unit = u'‰',
label=record,
lat = df['geo_meanLat'],
lon=df['geo_meanLon'],
archiveType='speleothem',
dropna=False,
verbose=False
)
if record == 'Qunf.Oman.2023': # Removing a large hiatus from the Qunf record
ens_series = ens_series.slice((2500,10000))
processed_ens_series = ens_series.slice(holocene_bounds).interp().standardize().detrend(method='savitzky-golay')
ens_series_list.append(ens_series)
processed_ens_series_list.append(processed_ens_series)
ens_dict[record] = pyleo.EnsembleGeoSeries(ens_series_list)
processed_ens_dict[record] = pyleo.EnsembleGeoSeries(processed_ens_series_list)
Loading 14 LiPD files
0%| | 0/14 [00:00<?, ?it/s]
14%|████████████ | 2/14 [00:00<00:02, 4.18it/s]
29%|████████████████████████ | 4/14 [00:00<00:01, 6.79it/s]
36%|██████████████████████████████ | 5/14 [00:00<00:01, 5.76it/s]
43%|████████████████████████████████████ | 6/14 [00:01<00:01, 5.87it/s]
50%|██████████████████████████████████████████ | 7/14 [00:01<00:01, 6.43it/s]
57%|████████████████████████████████████████████████ | 8/14 [00:01<00:01, 4.79it/s]
64%|██████████████████████████████████████████████████████ | 9/14 [00:01<00:01, 4.88it/s]
71%|███████████████████████████████████████████████████████████▎ | 10/14 [00:01<00:00, 5.33it/s]
79%|█████████████████████████████████████████████████████████████████▏ | 11/14 [00:01<00:00, 5.90it/s]
86%|███████████████████████████████████████████████████████████████████████▏ | 12/14 [00:02<00:00, 5.73it/s]
93%|█████████████████████████████████████████████████████████████████████████████ | 13/14 [00:02<00:00, 5.29it/s]
100%|███████████████████████████████████████████████████████████████████████████████████| 14/14 [00:02<00:00, 5.74it/s]
Loaded..
0%| | 0/14 [00:00<?, ?it/s]
Loading 1 LiPD files
0%| | 0/1 [00:00<?, ?it/s]
100%|█████████████████████████████████████████████████████████████████████████████████████| 1/1 [00:00<00:00, 12.38it/s]
Loaded..
7%|██████ | 1/14 [00:01<00:22, 1.71s/it]
Loading 1 LiPD files
0%| | 0/1 [00:00<?, ?it/s]
100%|█████████████████████████████████████████████████████████████████████████████████████| 1/1 [00:00<00:00, 3.06it/s]
100%|█████████████████████████████████████████████████████████████████████████████████████| 1/1 [00:00<00:00, 3.06it/s]
Loaded..
14%|████████████ | 2/14 [00:09<01:00, 5.08s/it]
Loading 1 LiPD files
0%| | 0/1 [00:00<?, ?it/s]
100%|█████████████████████████████████████████████████████████████████████████████████████| 1/1 [00:00<00:00, 12.05it/s]
Loaded..
21%|██████████████████ | 3/14 [00:10<00:38, 3.46s/it]
Loading 1 LiPD files
0%| | 0/1 [00:00<?, ?it/s]
100%|█████████████████████████████████████████████████████████████████████████████████████| 1/1 [00:00<00:00, 11.76it/s]
Loaded..
29%|████████████████████████ | 4/14 [00:12<00:27, 2.73s/it]
Loading 1 LiPD files
0%| | 0/1 [00:00<?, ?it/s]
100%|█████████████████████████████████████████████████████████████████████████████████████| 1/1 [00:00<00:00, 4.23it/s]
100%|█████████████████████████████████████████████████████████████████████████████████████| 1/1 [00:00<00:00, 4.21it/s]
Loaded..
36%|██████████████████████████████ | 5/14 [00:16<00:27, 3.11s/it]
Loading 1 LiPD files
0%| | 0/1 [00:00<?, ?it/s]
100%|█████████████████████████████████████████████████████████████████████████████████████| 1/1 [00:00<00:00, 6.20it/s]
100%|█████████████████████████████████████████████████████████████████████████████████████| 1/1 [00:00<00:00, 6.17it/s]
Loaded..
43%|████████████████████████████████████ | 6/14 [00:18<00:23, 2.94s/it]
Loading 1 LiPD files
0%| | 0/1 [00:00<?, ?it/s]
100%|█████████████████████████████████████████████████████████████████████████████████████| 1/1 [00:00<00:00, 7.94it/s]
100%|█████████████████████████████████████████████████████████████████████████████████████| 1/1 [00:00<00:00, 7.89it/s]
Loaded..
50%|██████████████████████████████████████████ | 7/14 [00:20<00:18, 2.70s/it]
Loading 1 LiPD files
0%| | 0/1 [00:00<?, ?it/s]
100%|█████████████████████████████████████████████████████████████████████████████████████| 1/1 [00:00<00:00, 2.91it/s]
100%|█████████████████████████████████████████████████████████████████████████████████████| 1/1 [00:00<00:00, 2.90it/s]
Loaded..
57%|████████████████████████████████████████████████ | 8/14 [00:27<00:22, 3.79s/it]
Loading 1 LiPD files
0%| | 0/1 [00:00<?, ?it/s]
100%|█████████████████████████████████████████████████████████████████████████████████████| 1/1 [00:00<00:00, 4.75it/s]
100%|█████████████████████████████████████████████████████████████████████████████████████| 1/1 [00:00<00:00, 4.74it/s]
Loaded..
64%|██████████████████████████████████████████████████████ | 9/14 [00:30<00:18, 3.78s/it]
Loading 1 LiPD files
0%| | 0/1 [00:00<?, ?it/s]
100%|█████████████████████████████████████████████████████████████████████████████████████| 1/1 [00:00<00:00, 6.35it/s]
100%|█████████████████████████████████████████████████████████████████████████████████████| 1/1 [00:00<00:00, 6.31it/s]
Loaded..
71%|███████████████████████████████████████████████████████████▎ | 10/14 [00:33<00:13, 3.50s/it]
Loading 1 LiPD files
0%| | 0/1 [00:00<?, ?it/s]
100%|█████████████████████████████████████████████████████████████████████████████████████| 1/1 [00:00<00:00, 7.44it/s]
100%|█████████████████████████████████████████████████████████████████████████████████████| 1/1 [00:00<00:00, 7.39it/s]
Loaded..
79%|█████████████████████████████████████████████████████████████████▏ | 11/14 [00:36<00:09, 3.20s/it]
Loading 1 LiPD files
0%| | 0/1 [00:00<?, ?it/s]
100%|█████████████████████████████████████████████████████████████████████████████████████| 1/1 [00:00<00:00, 4.85it/s]
100%|█████████████████████████████████████████████████████████████████████████████████████| 1/1 [00:00<00:00, 4.83it/s]
Loaded..
86%|███████████████████████████████████████████████████████████████████████▏ | 12/14 [00:40<00:06, 3.43s/it]
Loading 1 LiPD files
0%| | 0/1 [00:00<?, ?it/s]
100%|█████████████████████████████████████████████████████████████████████████████████████| 1/1 [00:00<00:00, 4.50it/s]
100%|█████████████████████████████████████████████████████████████████████████████████████| 1/1 [00:00<00:00, 4.49it/s]
Loaded..
93%|█████████████████████████████████████████████████████████████████████████████ | 13/14 [00:44<00:03, 3.66s/it]
Loading 1 LiPD files
0%| | 0/1 [00:00<?, ?it/s]
100%|█████████████████████████████████████████████████████████████████████████████████████| 1/1 [00:00<00:00, 14.57it/s]
Loaded..
100%|███████████████████████████████████████████████████████████████████████████████████| 14/14 [00:45<00:00, 2.94s/it]
100%|███████████████████████████████████████████████████████████████████████████████████| 14/14 [00:45<00:00, 3.26s/it]
Plotting each record and its ensemble for QA/QC:
for label,series in series_dict.items():
ens = ens_dict[label]
fig,ax = ens.common_time().plot_envelope()
series.plot(ax=ax)
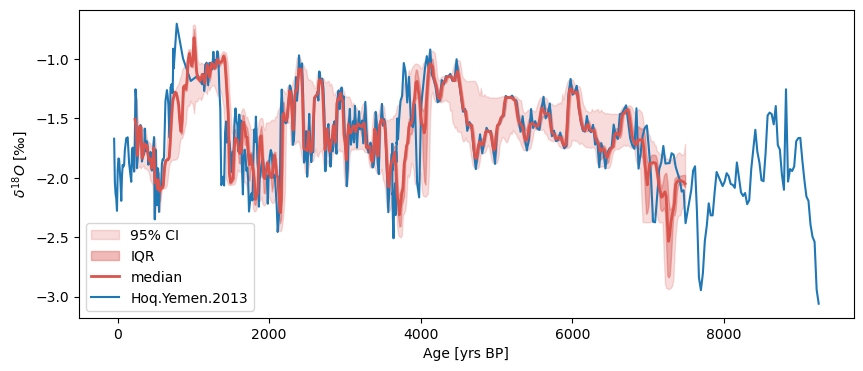
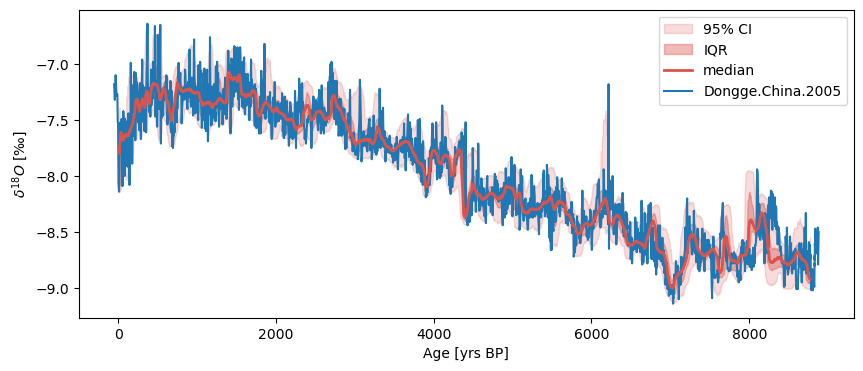
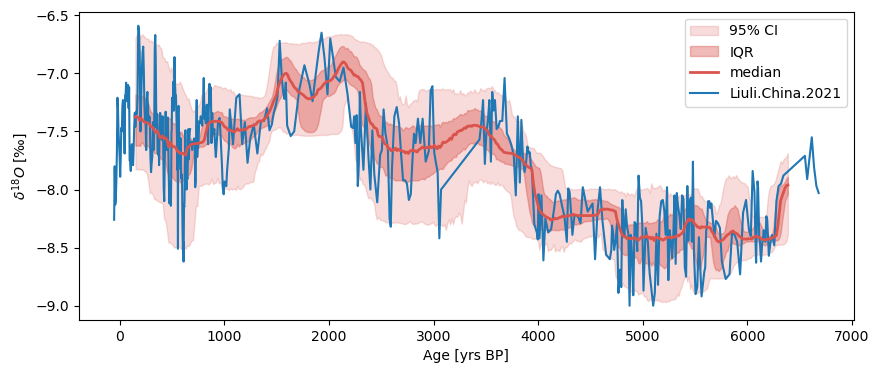
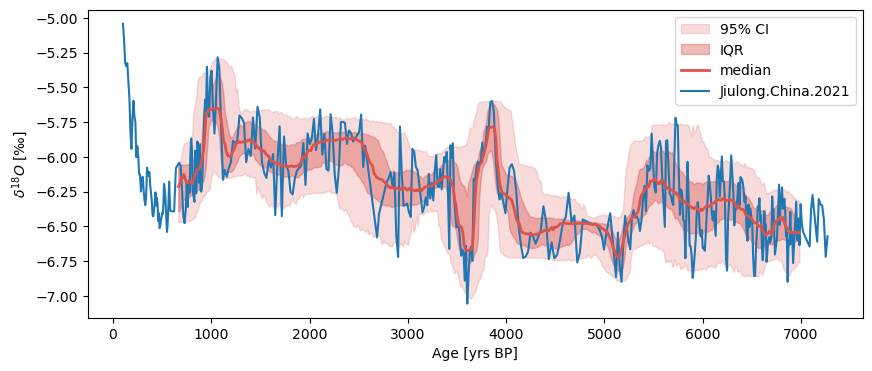
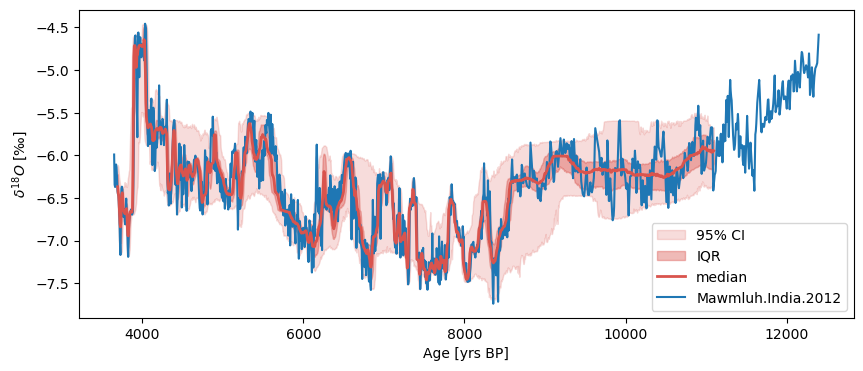
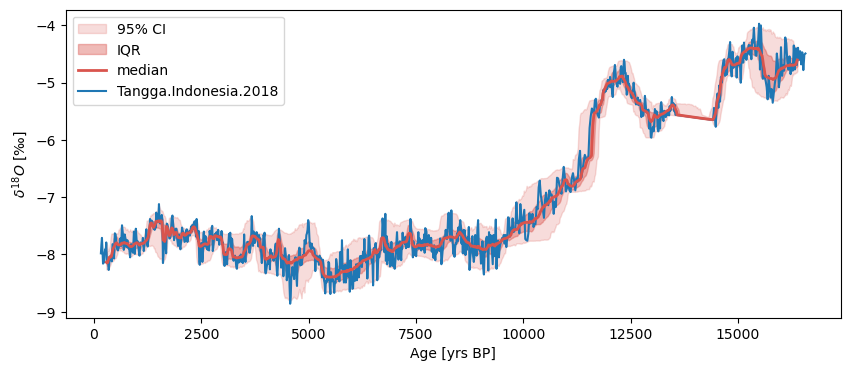
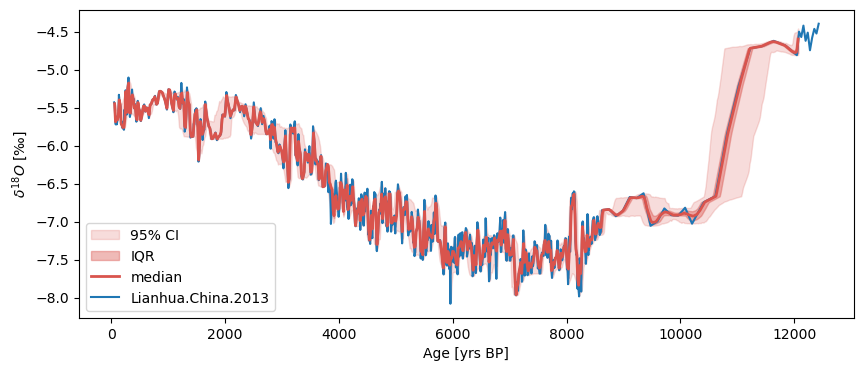
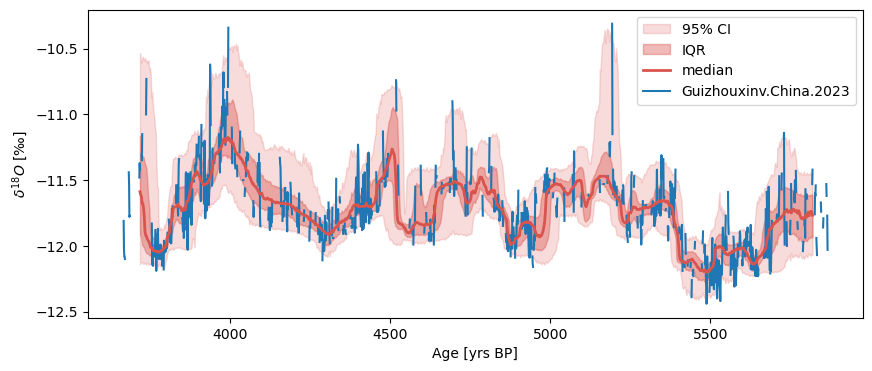
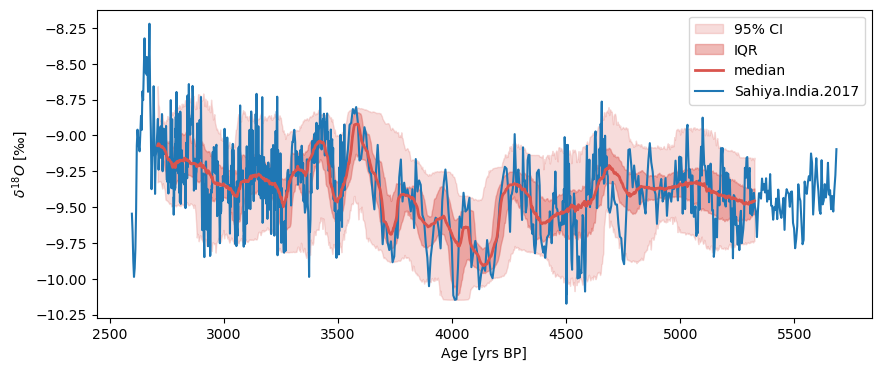
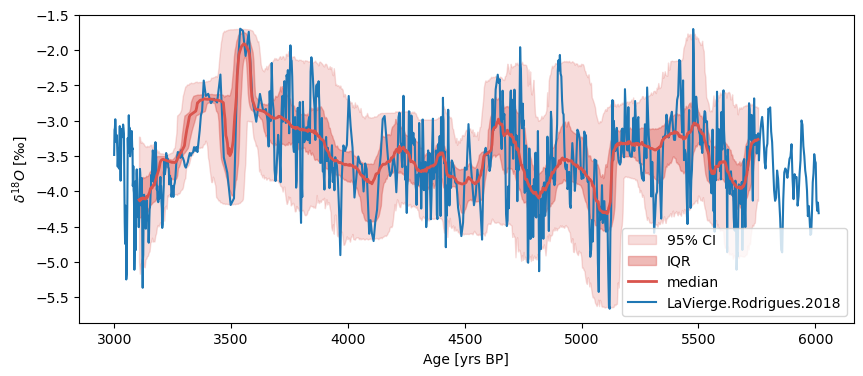
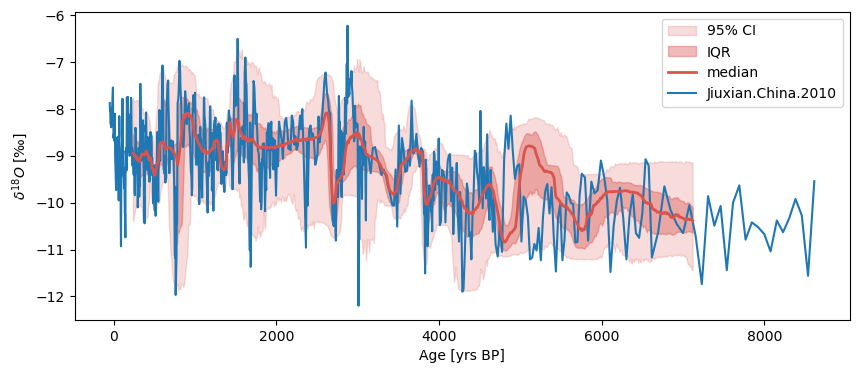
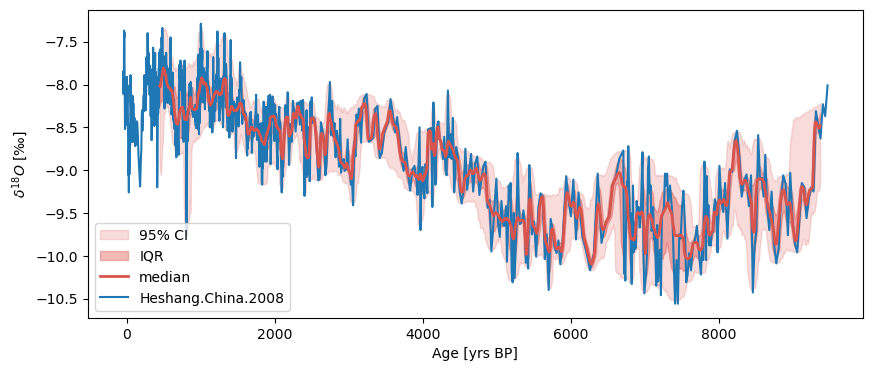
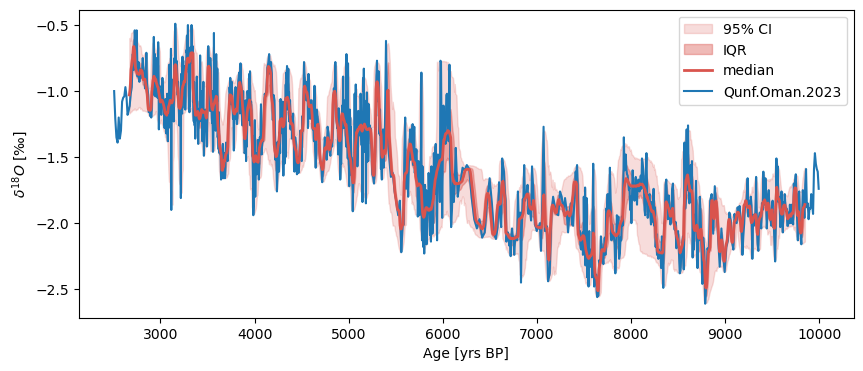
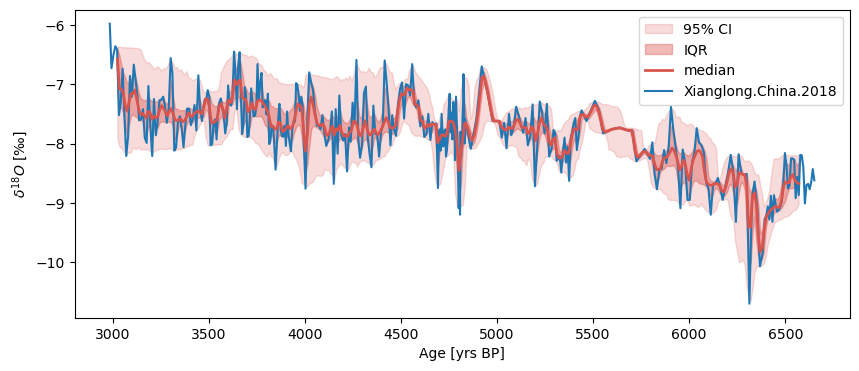
Saving the data:
with open('../../data/pickle/series_dict.pkl','wb') as handle:
pickle.dump(series_dict,handle)
with open('../../data/pickle/ens_dict.pkl','wb') as handle:
pickle.dump(ens_dict,handle)
Plotting each processed record and its ensemble for QA/QC:
for label,series in processed_series_dict.items():
ens = processed_ens_dict[label]
fig,ax = ens.common_time().plot_envelope()
series.plot(ax=ax)
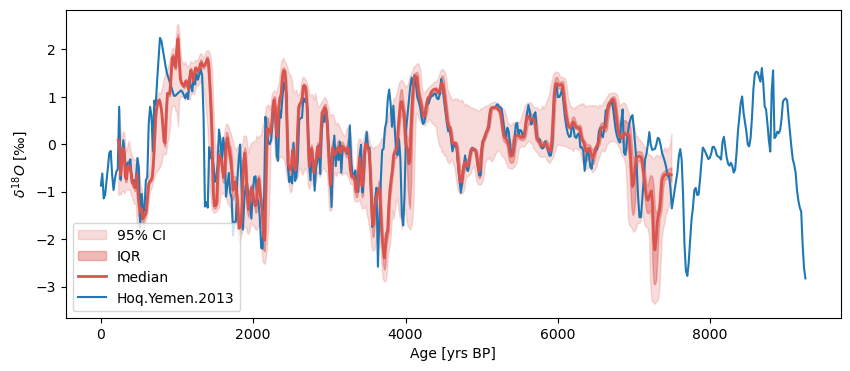
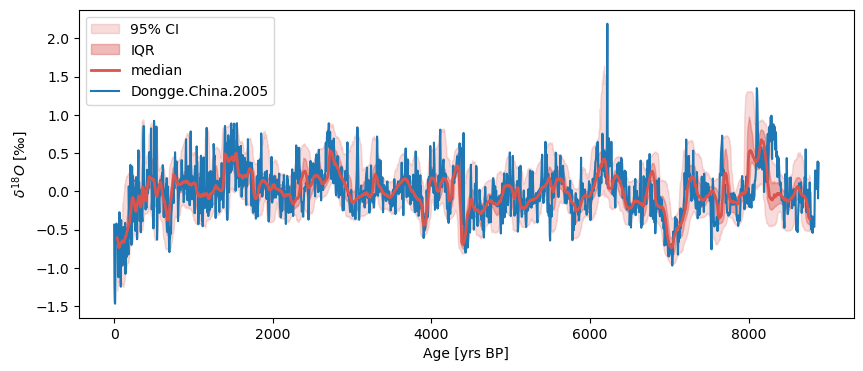
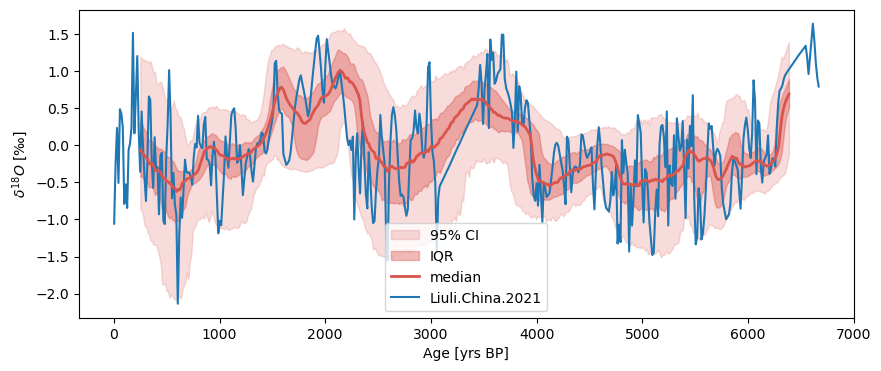
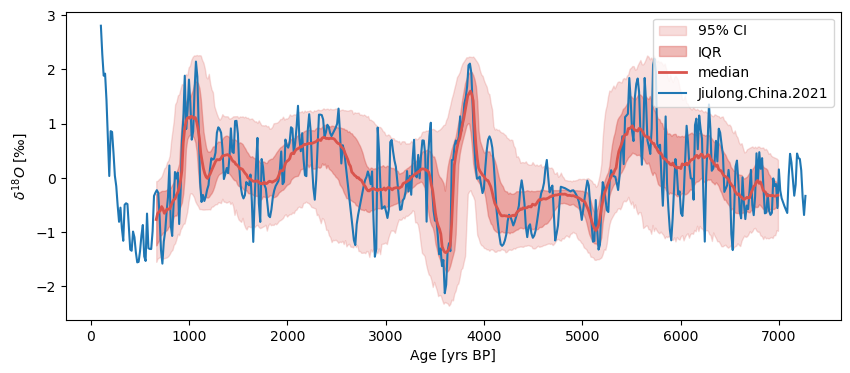
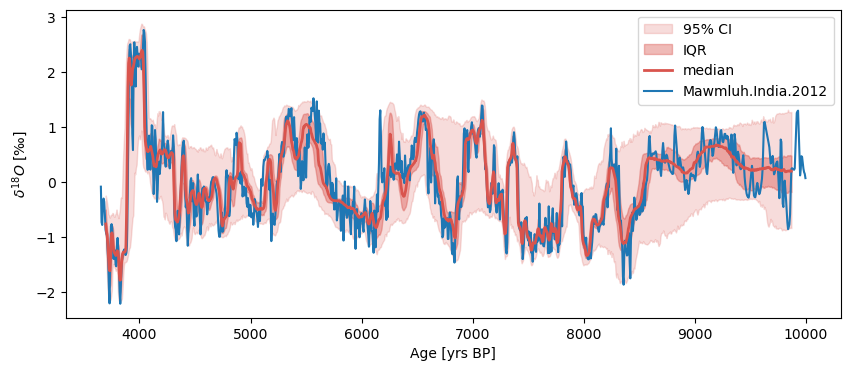
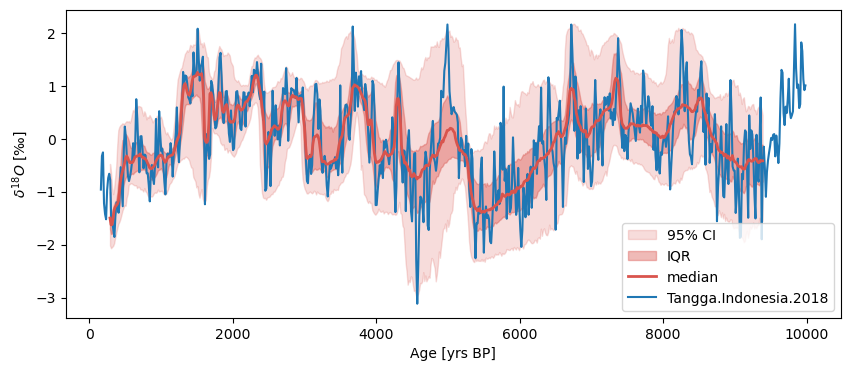
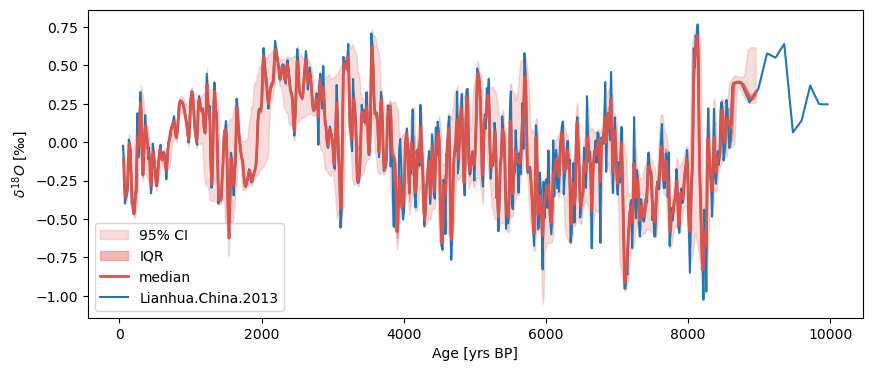
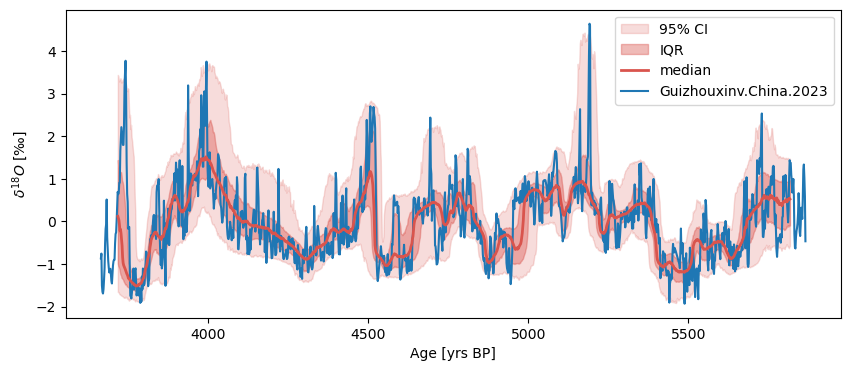
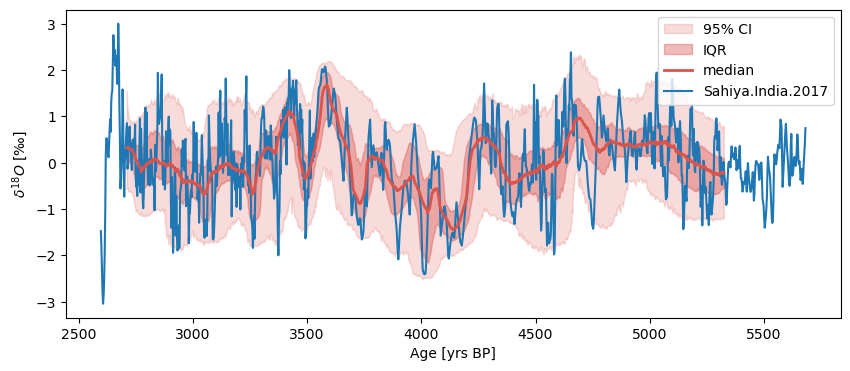
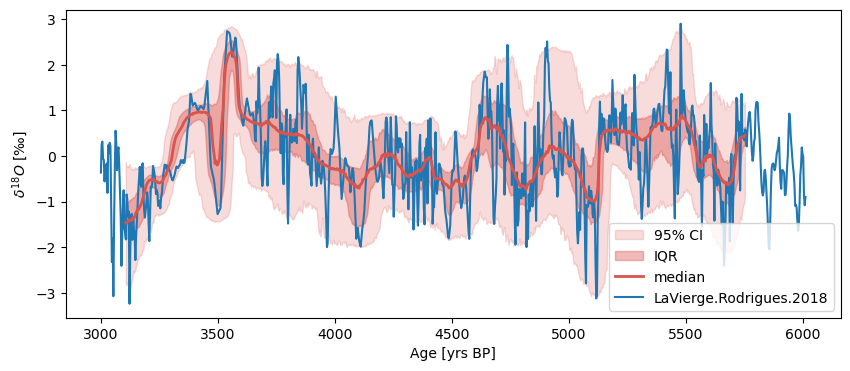
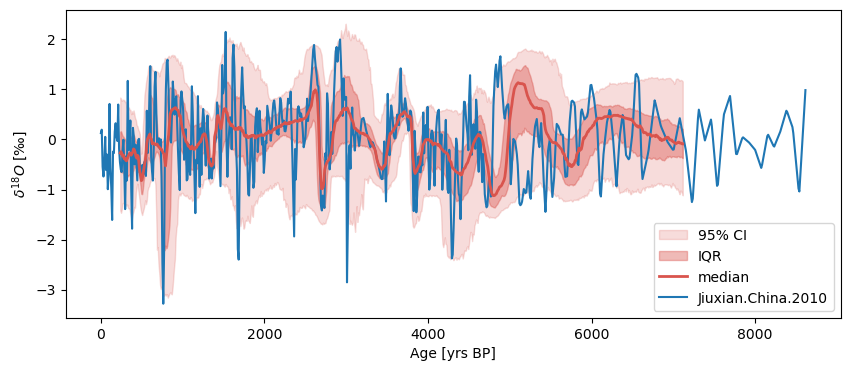
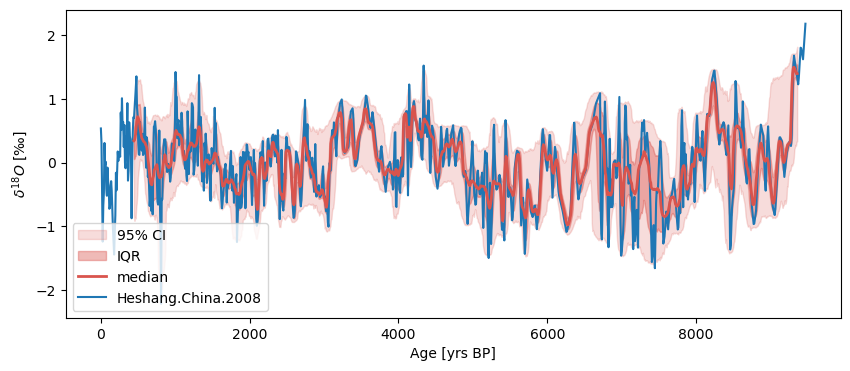
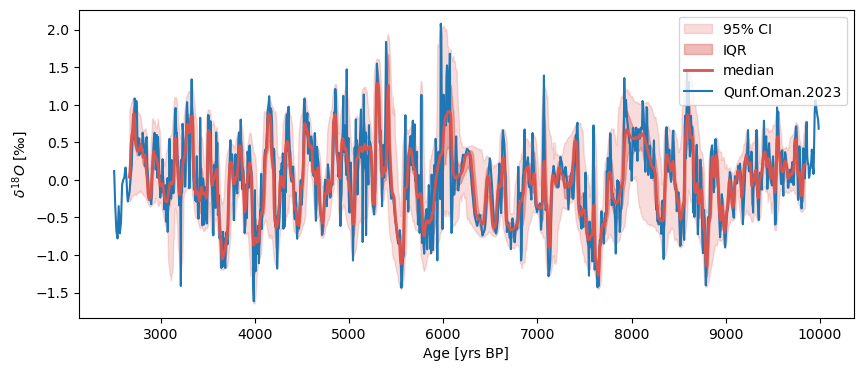
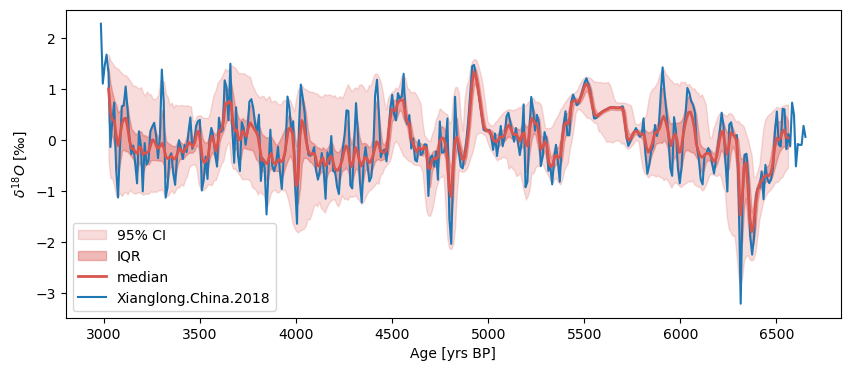
Saving the processed records and their ensembles:
with open('../../data/pickle/preprocessed_series_dict.pkl','wb') as handle:
pickle.dump(processed_series_dict,handle)
with open('../../data/pickle/preprocessed_ens_dict.pkl','wb') as handle:
pickle.dump(processed_ens_dict,handle)